Mounting Components
Many tests, regardless of framework or type, follow a similar format: Arrange, Act, and Assert. This pattern "Arrange, Act, Assert" was first coined in 2001 by Bill Wilke and is explained thoroughly in his blog post "3A - Arrange, Act, Assert".
When it comes to component testing, mounting your component is where we Arrange our component under test. It is analogous to visiting a page in an end-to-end test.
What is the Mount Function?
We ship a mount
function for each front-end framework Cypress supports, which
is imported from the cypress
package. It is responsible for rendering
components within Cypress's sandboxed iframe and handling and framework-specific
cleanup.
import { mount } from 'cypress/vue'
Vue 2 projects will import the mount
function from cypress/vue2
like so:
import { mount } from 'cypress/vue2'
cy.mount()
Anywhere
Using While you can use the mount
function in your tests, we recommend using
cy.mount()
, which is added as a
custom command in the
cypress/support/component.js file:
import { mount } from 'cypress/vue'
Cypress.Commands.add('mount', mount)
This allows you to use cy.mount()
in any component test without having to
import the framework-specific mount command.
You can customize cy.mount
to fit your needs. For instance, if you are using
plugins or other global app-level setups in your Vue app, you can configure them
here. For more info, see the
Customizing cy.mount() guide for Vue.
Your First Component Test
Now that you have a component let's write a spec that mounts the component.
To get started, create a spec file in the same directory as the Stepper.vue
component and name it Stepper.cy.js. Then paste the following into it:
import Stepper from './Stepper.vue'
describe('<Stepper>', () => {
it('mounts', () => {
cy.mount(Stepper)
})
})
Here, we have a single test that ensures that our component mounts.
If you already have a component that you want to create a spec for, we can scaffold the spec for you via the Cypress App.
Click on "+ New Spec", select "Create from component", and choose your component from the list.
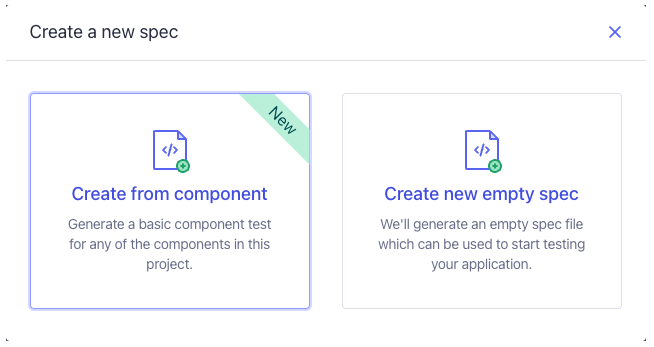
Create from component card
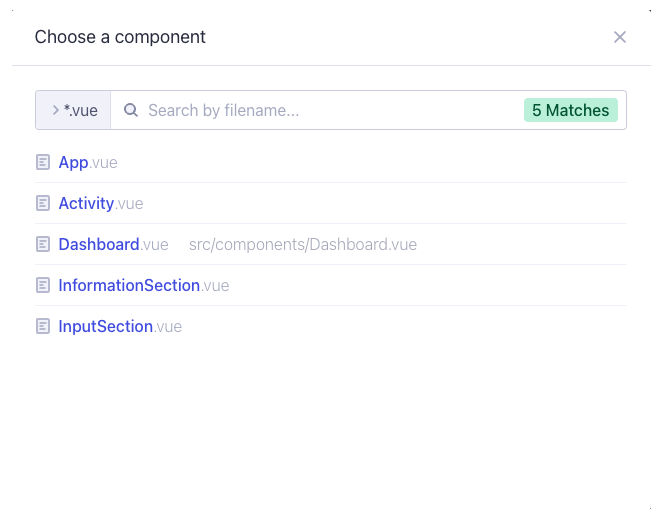
Create from component list
If you're coming from Vue Test Utils, please note that the return value of
mount
is not used. Cypress Component tests can and should be agnostic to the framework internals and accessing thewrapper
that Vue Test Utils rely on is rarely necessary.
Running the Test
Now it's time to see the test in action. Open up Cypress if it is not already running:
npx cypress open --component
The
--component
flag will launch us directly into component testing
And launch the browser of your choice. In the spec list, click on Stepper.cy.js and you will see the stepper component mounted in the test area.
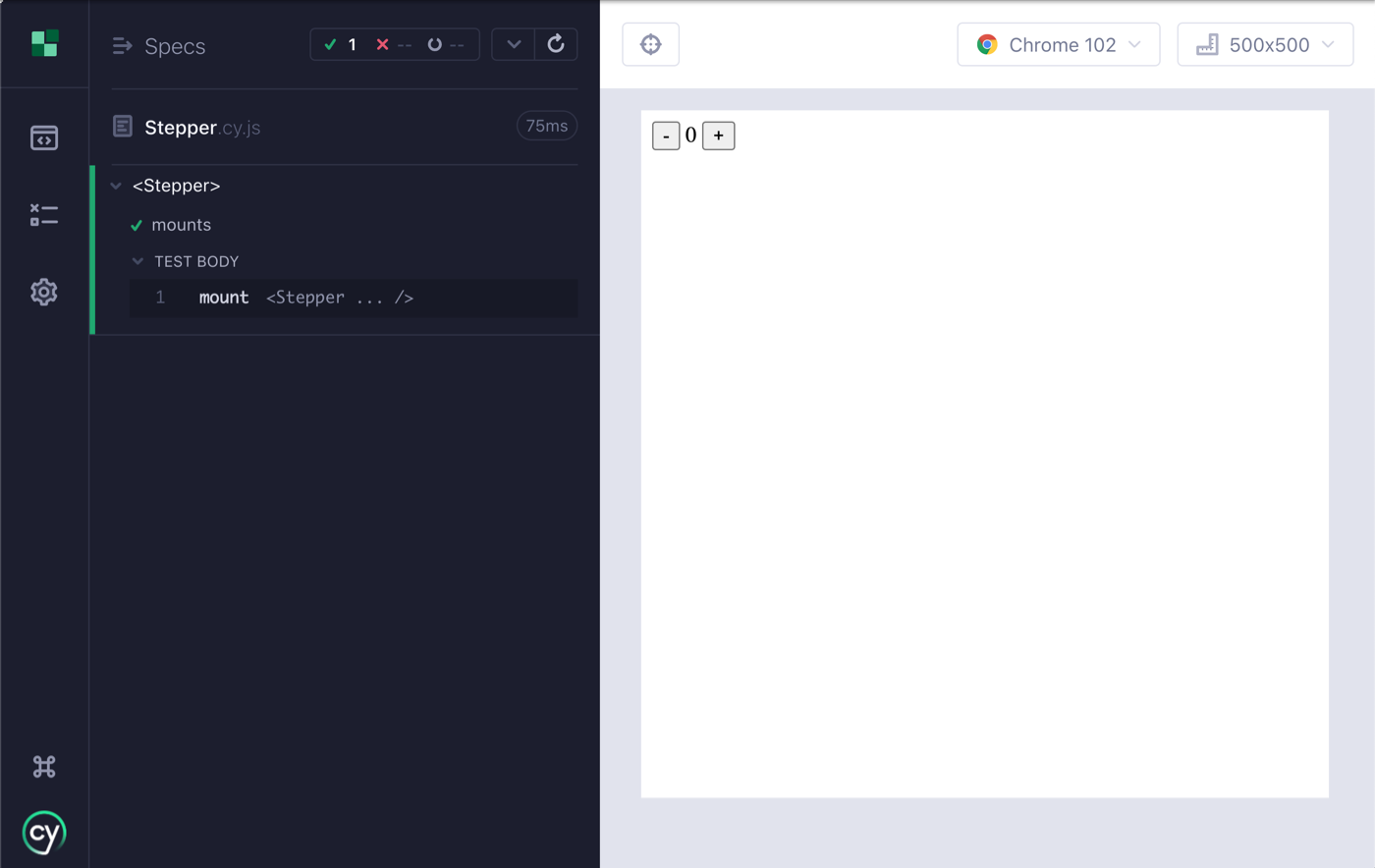
Stepper Mount Test
A basic test that mounts a component in its default state is an excellent way to start testing. Since Cypress renders your component in a real browser, you have a lot of advantages, such as seeing that the component renders as it should, interacting with the component in the test runner, and using the browser dev tools to inspect and debug both your tests and the component's code.
Feel free to play around with the Stepper
component by interacting with the
increment and decrement buttons.
Optional JSX Support
The mount command supports the Vue Test Utils object syntax, but it can also be used with Vue's JSX syntax (provided that you've configured your bundler to support transpiling JSX or TSX files).
The object syntax for the mount function is identical to the Vue Test Utils version you'd use with your application's version of Vue.
The rest of this guide will show tests in Vue Test Utils syntax and JSX.
Cypress and Testing Library
While we don't use Testing Library in this guide, many people might wonder if it is possible to do so with Cypress and the answer is yes!
Cypress loves the Testing Library project. We use Testing Library internally, and our philosophy aligns closely with Testing Library's ethos and approach to writing tests. We strongly endorse their best practices.
In particular, if you're looking for more resources to understand how we recommend you approach testing your components, look to:
For fans of
Testing Library,
you'll want to install @testing-library/cypress
instead of the
@testing-library/react
package.
npm i -D @testing-library/cypress
The setup instructions are the same for E2E and Component Testing. Within your component support file, import the custom commands.
// cypress/support/component.js
// cy.findBy* commands will now be available.
// This calls Cypress.Commands.add under the hood
import '@testing-library/cypress/add-commands'
For TypeScript users, types are packaged along with the Testing Library package. Refer to the latest setup instructions in the Testing Library docs.
Next Steps
Now that we have our component mounted, next we will learn how to write tests against it.